React Conditional Rending Components
When writing react code avoid using conditional components like so:
export default function Scores({score}) {
return (
<>
{score < 30
? "π"
: score < 70 && score >= 30
? "π"
: "π"}
</>
)
}
They are difficult to read and very difficult to scale in case you need to cover yet more cases.
Instead you can build your own conditional rendering components that would look something like this:
export default function Scores({ score }) {
return (
<Switch>
<Case condition={score < 30}>π</Case>
<Case condition={score < 70 && score >= 30}>π</Case>
<Case condition={score >= 70}>WOW</Case>
<Default>π</Default>
</Switch>
)
}
As you can easily see this code looks easy to read and scale. To build these components here’s a headstart:
const Switch = ({ children }) => {
let matched = false
let defaultCase = null
React.Children.forEach(children, (child) => {
if (!matched && child.type === Case) {
const { condition } = child.props
const conditionResult = Boolean(condition)
if (conditionResult) {
matched = child
}
} else if (!defaultCase && child.type === Default) {
defaultCase = child
}
})
return matched ?? defaultCase ?? null
}
const Case = ({ children }) => children
const Default = ({ children }) => children
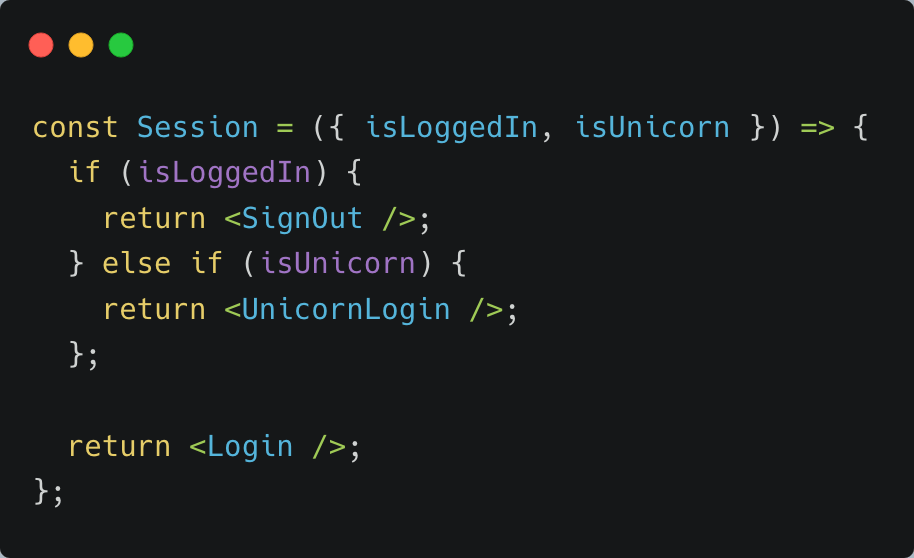